次の担当はFlyweightパターン。
同じものを共有して無駄をなくすパターンです。
どこの無駄を無くすのかというとメモリの使用量です。
インスタンスをできるだけ共有して無駄にnewしないことでインスタンスを
沢山作らせない→メモリの使用量を減らすということです。
クラス図
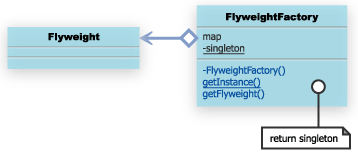
サンプルコード
メリット
オブジェクトを生成する処理をクライアント側から隠蔽することができます。
インスタンスを使いまわすのでメモリ消費量が抑えられる。
newの回数が減らせるのでプログラムのパフォーマンスもあげられる。
デメリット
Flyweight Objectはどこから呼ばれるかわからないので状態を持ってはいけない。
システム全体で、使いまわすインスタンスの把握が必要。
一度
ガベージコレクションされることがないため、場合によっては明示的に
から削除する必要がある。
使いどころ
Flyweight パターンを採用すべき典型的な例は、不変なクラスを扱う場合。
不変なクラスとはインスタンスが生成された後にそのインスタンスの状態が変化しないようなクラス。
対象が不変でないクラスの場合は、その状態が変更されている場合に再利用できないのでこのパターンに適しません。
同じものを共有して無駄をなくすパターンです。
どこの無駄を無くすのかというとメモリの使用量です。
インスタンスをできるだけ共有して無駄にnewしないことでインスタンスを
沢山作らせない→メモリの使用量を減らすということです。
クラス図
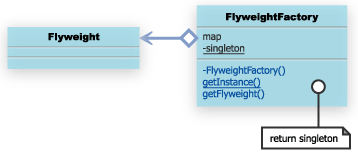
サンプルコード
class BigChar { private $charName; // 文字の名前 private $fontdata; // 大きな文字を表す文字列 public function __construct($charName) { $this->charName = $charName; try { $fileName = "big" . $this->charName . ".txt"; if (!file_exists($fileName)) { throw new Exception(); } $this->fontdata = implode("", file($fileName)); } catch (Exception $e) { $this->fontdata = $this->charName . "?"; } } public function prints() { echo $this->fontdata; } } class BigCharFactory { private $pool = array(); private static $singleton; private function __construct(){} // 外からnewさせない public static function getInstance() { if (!is_object(BigCharFactory::$singleton)) { BigCharFactory::$singleton =& new BigCharFactory(); } return BigCharFactory::$singleton; } public function getBigChar($charName) { $bc = isset($this->pool[$charName]) ? $this->pool[$charName] : null; if ($bc == null) { $bc = new BigChar($charName); $this->pool[$charName] = $bc; } return $bc; } } class BigString { private $bigChars = array(); public function __construct($string) { $factory = BigCharFactory::getInstance(); for ($i = 0; $i < strlen($string); $i++) { $this->bigChars[$i] = $factory->getBigChar(substr($string, $i, 1)); } } public function prints() { for ($i = 0; $i < count($this->bigChars); $i++) { $this->bigChars[$i]->prints(); } } } if ($argc < 2) { echo "Usage: php flyweight.php digits\n"; echo "Example: php flyweight.php 1212123\n"; exit; } $bs = new BigString($argv[1]); $bs->prints();実行結果
$ php self_sample.php 123 ......##........ ..######........ ......##........ ......##........ ......##........ ......##........ ..##########.... ................ ....######...... ..##......##.... ..........##.... ......####...... ....##.......... ..##............ ..##########.... ................ ....######...... ..##......##.... ..........##.... ......####...... ..........##.... ..##......##.... ....######...... ................
利用者は Flyweight
クラスにあたるインスタンスを取得する場合に、直接その
クラスのコンストラクタを呼び出す代わりに BigCharFactory#
getBigChar()
にアクセスする。 一方、呼び出された
BigChar
Factory
オブジェクトは、状況に
応じて振る舞いを変える。
その時点で対象のインスタンスが生成されていない場合- 対象のインスタンスを新たに生成する。
- 生成したインスタンスをプールする(言い換えると、メンバのコンテナオブジェクトに格納する)。
- 生成されたインスタンスを返す。
- 対象のインスタンスをプールから呼び出す。
- 対象のインスタンスを返す。
このように BigChar
Factory
では状況によって処理は違うけれど、利用者側が
得る結果は全く同じなので、利用者は BigChar
Factory
の内部構造を意識せず
に使うことが出来る。
メリット
オブジェクトを生成する処理をクライアント側から隠蔽することができます。
インスタンスを使いまわすのでメモリ消費量が抑えられる。
newの回数が減らせるのでプログラムのパフォーマンスもあげられる。
デメリット
Flyweight Objectはどこから呼ばれるかわからないので状態を持ってはいけない。
システム全体で、使いまわすインスタンスの把握が必要。
一度
FlyweightFactory
に保存されたインスタンスは、たとえ不要になった場合でもガベージコレクションされることがないため、場合によっては明示的に
FlyweightFactory
から削除する必要がある。
使いどころ
Flyweight パターンを採用すべき典型的な例は、不変なクラスを扱う場合。
不変なクラスとはインスタンスが生成された後にそのインスタンスの状態が変化しないようなクラス。
対象が不変でないクラスの場合は、その状態が変更されている場合に再利用できないのでこのパターンに適しません。
My developer is trying to persuade me to move to .net from PHP. I have always disliked the idea because of the expenses. But he's tryiong none the less. I've been using Movable-type on various websites for about a year and am nervous about switching to another platform. I have heard fantastic things about blogengine.net. Is there a way I can transfer all my wordpress posts into it? Any help would be really appreciated!
The holy Virgin and the fine God take care of 'em! He had had almost all nighttime since half-past fifteen to consider just what next was most fundamental for them to appreciate, along with a seriously complex request it was, you shall see.